Description
Command is a behavioral design pattern, used to encapsulate actions to be performed and their parameters. It defines a command as an object sent from the client to the recipient, which allows for such functionalities as: command queuing, history checking (even with “undo” operation), etc.
Usage
Command Pattern is commonly used in REST API by many large applications. (example API: Allegro).
UML Diagram
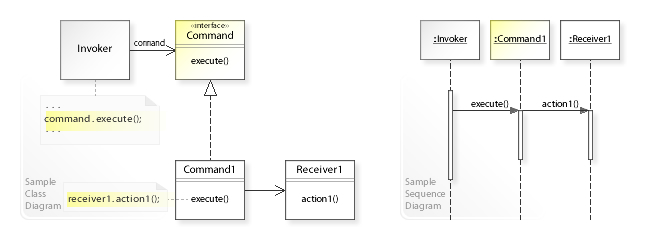
PHP code implementation / example (GitHub)
…
Real life example uncase
Request:
PUT /business-resource/{resource-identifier}/{command-type}-commands/{uuid-command-identifier}
Body:
{
// input data relevant for the command, e.g.:
“input”: {
"buyNowPrice": {
"amount": "122",
"currency": "PLN"
}
}
}
Response:
201 Created
{
“id”: “9b84e1bc-5341-45e7-837e-4250720e606f”,
“input”: {
"buyNowPrice": {
"amount": "122",
"currency": "PLN"
}
},
“output”: {
"status" : "RUNNING",
"errors" : []
// other return data relevant for the command
}
}
For example, a resource enabling the execution of the price change operation is:
PUT /offers/12345/change-price-commands/84c16171-233a-42de-8115-1f1235c8bc0f
In executing a request for such resource, you should add the price change command identifier 84c16171-233a-42de-8115-1f1235c8bc0f, to the set of actions to be performed on the offer indicated by the identifier = 12345.
You should remember that we use the http PUT method instead of the POST method for these resources, which means that it is the client to indicate (send) an identifier for the command.